Introduction:
In the realm of software development, optimizing data retrieval and storage is crucial for performance. One powerful technique that aids in this optimization is Momento Cache. In this technical blog, we will delve into the concept of Momento Cache, its significance, and provide beginners with practical steps on how to integrate and get started with it in their projects.
Understanding Momento Cache:
Momento Cache is a design pattern that enables the efficient storage and retrieval of the state of an object or system at a specific point in time. By caching snapshots of the system's state, developers can reduce the need for repetitive, time-consuming computations or database queries, ultimately enhancing performance.
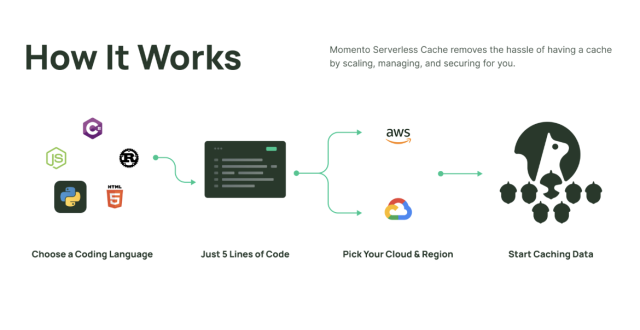
Getting Started:
1. Identify Cached Objects:
Determine the objects or data in your application that can benefit from caching. These could be frequently accessed database queries, computations, or any data that remains relatively static over short periods.
2. Choose a Caching Strategy:
- Decide on the caching strategy that best suits your application's requirements. Common strategies include Time-based caching, Least Recently Used (LRU) caching, and Dependency-based caching.
- Each strategy has its strengths and is suitable for specific scenarios.
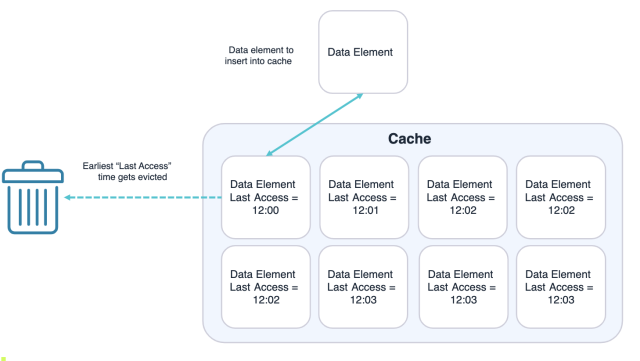
3. Integrate a Caching Library:
- Explore and choose a caching library that aligns with your programming language and application architecture.
- Popular choices include Redis, Memcached, or even built-in caching modules for frameworks like Django or Rails.
4. Implement Caching in Code:
- Begin integrating caching into your codebase. For example, if using a framework like Django, leverage its caching middleware.
- If you're working with a custom solution, use the caching library to store and retrieve cached data.
- Implement cache expiration policies to ensure data consistency.
from django.core.cache import cache
def get_data_from_database():
# Simulate a time-consuming database query
# ...
pass # Replace with actual database query logic
def get_data():
# Check if data is in the cache
data = cache.get('cached_data')
if data is None:
# If not, fetch data from the database
data = get_data_from_database()
# Store data in the cache with a timeout of 300 seconds (5 minutes)
cache.set('cached_data', data, 300)
return data
5. Handle Cache Invalidation:
- Plan for cache invalidation to ensure that outdated or stale data doesn't persist in the cache.
- This can be triggered based on events such as data updates or deletions.
6. Monitor and Optimize:
- Regularly monitor your application's performance using metrics and logging. Adjust caching strategies and expiration times based on usage patterns and evolving application needs.
- This iterative optimization is crucial for maintaining a well-balanced system.
Conclusion:
Momento Cache is a powerful tool in a developer's arsenal for optimizing data handling and improving application performance. By following these steps and integrating caching into your projects, you can strike a balance between resource efficiency and data consistency, creating a smoother and more responsive user experience . Experiment, iterate, and enjoy the performance benefits that Momento Cache brings to your applications.